Built-in Data Types
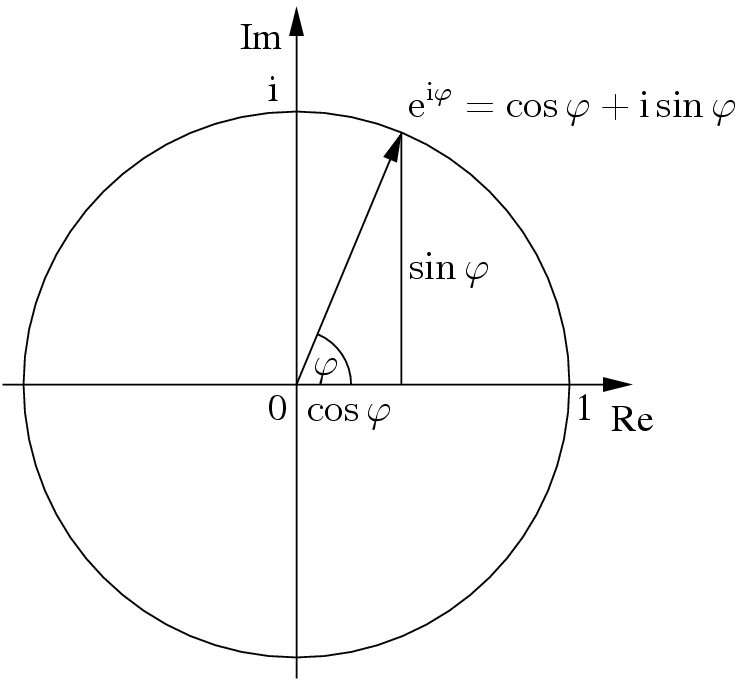
Just like any other programming languages, Go has a number of built-in data types:
Basic Data types
- bool
- string
- (u)int, (u)int8, (u)int16, (u)int32, (u)int64, uintptr
- u means unsigned
- uintptr means pointer
- byte (8 bits)
- rune (32 bits), similar to char in C
- float32, float64
- complex64, complex128
Go is one of few languages that support complex numbers which is widely used in computational applications.
1 | func complex_num() { |
We could use the complex number to play with the beautiful Euler formula
1 | func euler(){ |
It will print out results below, which is really close to 0.
1 | (0+1.2246467991473515e-16i) |
The trailing imaginary number is due to the float precision, we could get rid of it by using fmt.Printf
1 | func euler() { |
It will print out the following
1 | (0.000+0.000i) |
Type Conversion
There’s no implicit type conversion in Go, all convertions are explicit.
The example below will report type errors during compilation.
1 | func triangle() { |
In stead, we have to explicitly claim a, b, c
are floats
1 | func triangle() { |